Overview
This blog in the form of documentation provides a comprehensive guide on automating the process of extracting Google Play Store data, parsing it, and integrating it into Mixpanel using Node.js. By following these steps, you can streamline the process of analyzing app data and use Mixpanel for advanced analytics and insights.
The steps include setting up Google Cloud Storage, fetching data, converting it into a usable format, and sending the data to Mixpanel.
Prerequisites
To complete this integration, you will need:
- A Google Cloud Platform account.
- Google Play Console access with the appropriate API permissions.
- A Mixpanel account for tracking and analyzing events.
- Node.js installed on your machine
Step 1: Enable Google Play Developer API
The Google Play Developer API allows you to programmatically access Google Play Store data.
Here’s how to enable it:
- Open the Google Cloud Console (https://console.cloud.google.com).
- Go to APIs & Services > Library (https://console.cloud.google.com/apis/library).
- Search for "Google Play Developer API"
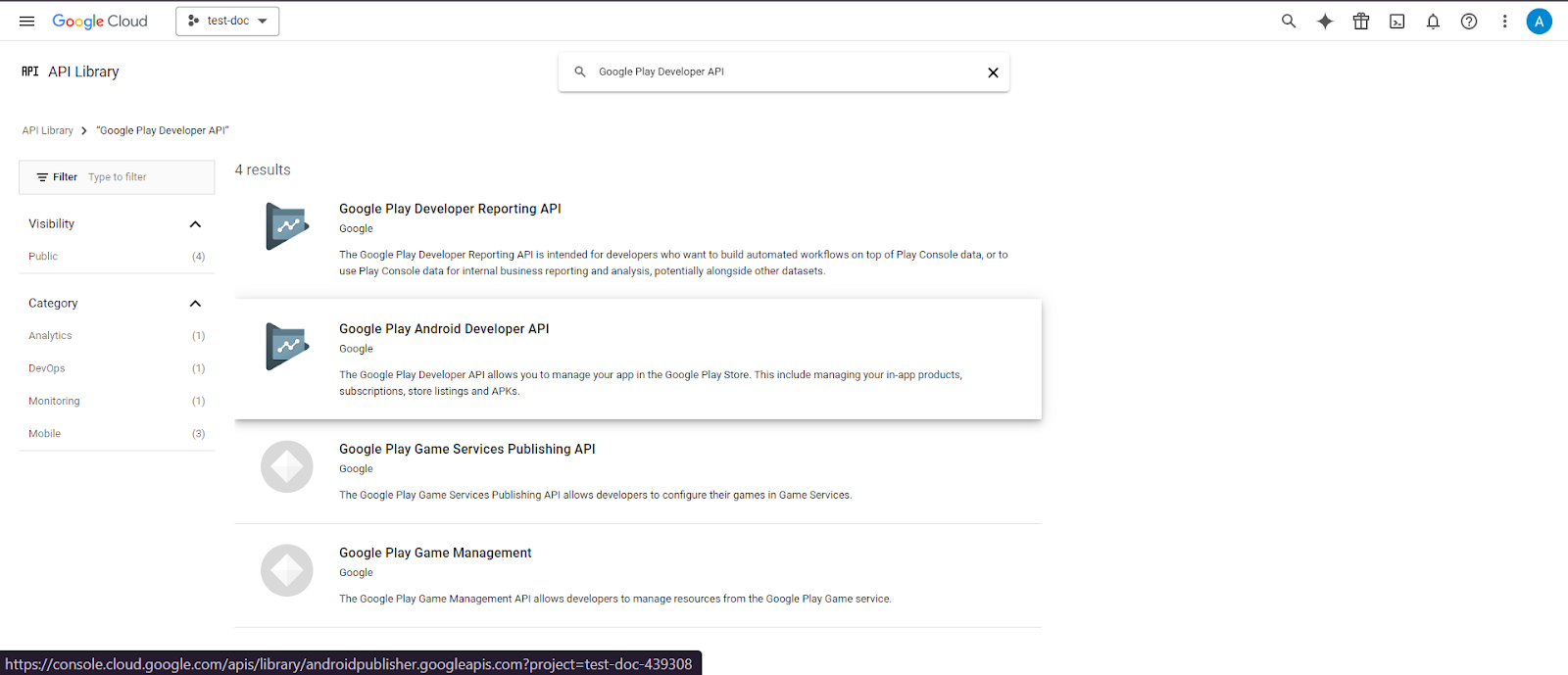
- Click Enable to activate the API.
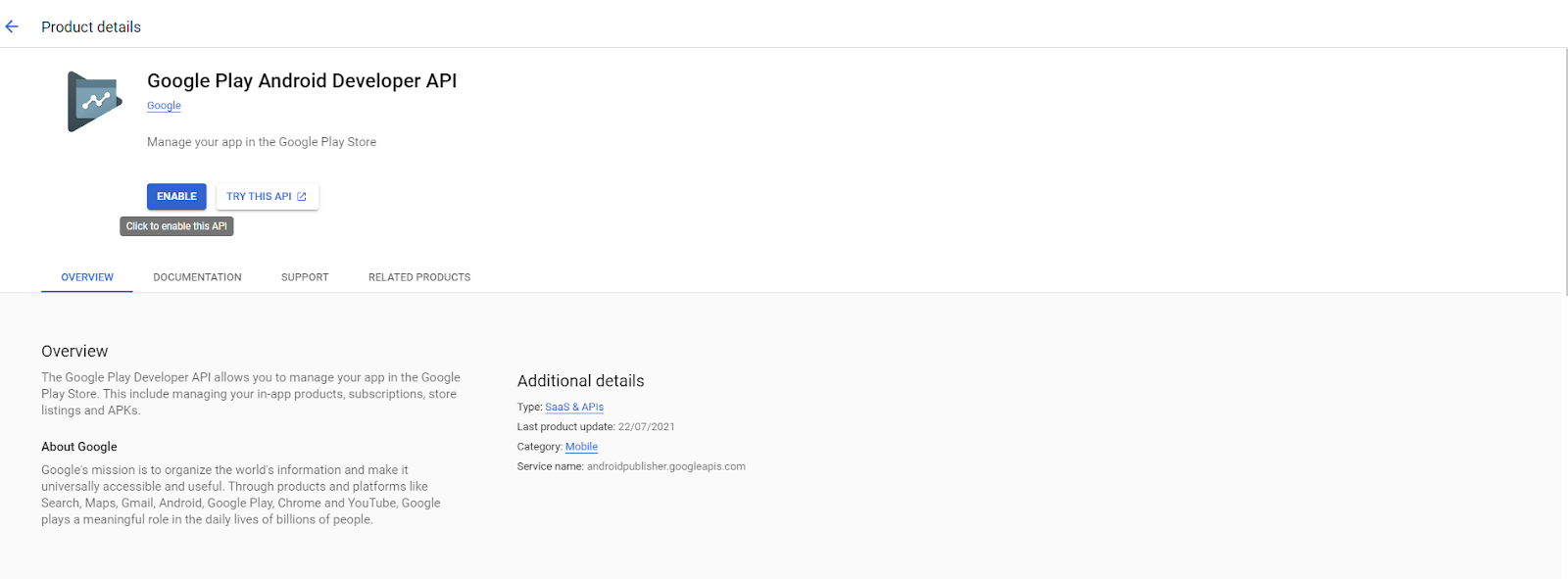
Enabling this API allows access to Google Play Store data through Google Cloud services, which is essential for automating data extraction.
Step 2: Create a Service Account
You will need a service account to access Google Cloud Storage. Follow these steps:
- Go to the Google Cloud Console (https://console.cloud.google.com/iam-admin/serviceaccounts).
- Navigate to IAM & Admin > Service Accounts (https://console.cloud.google.com/iam-admin/serviceaccounts).
- Click Create Service Account.
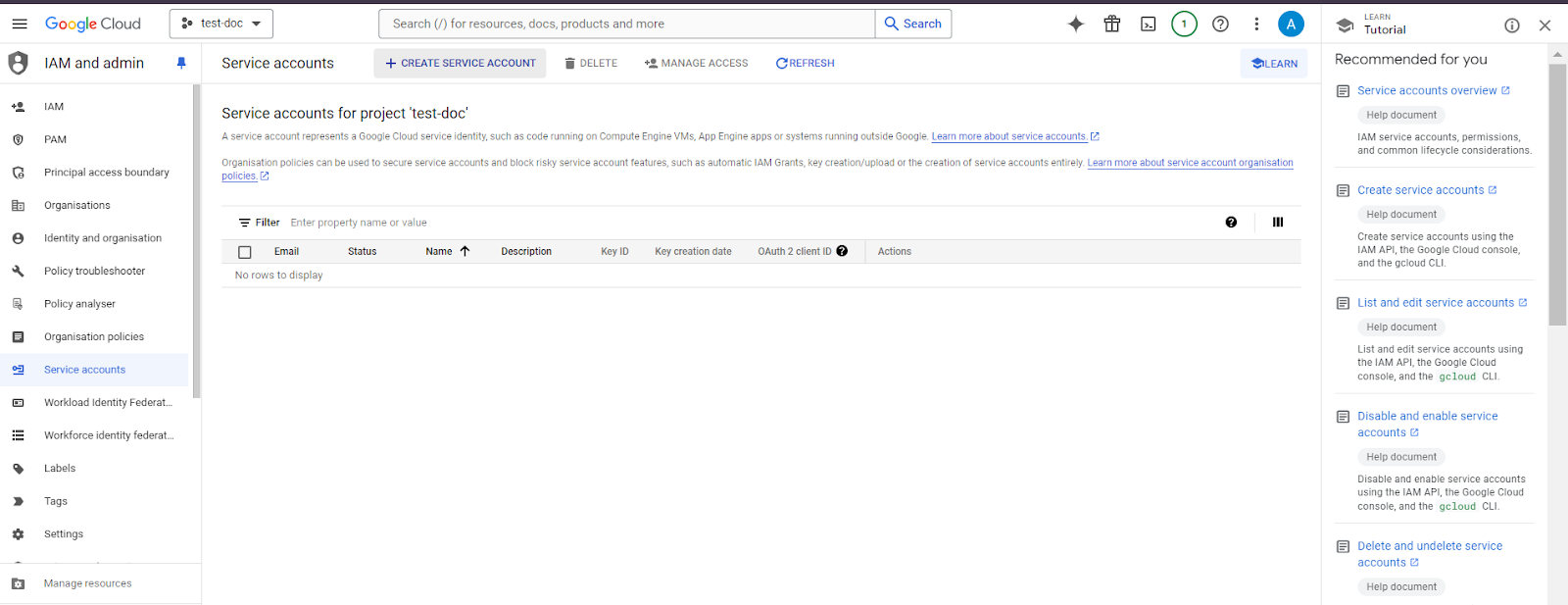
- Enter a name for your service account and click Create.
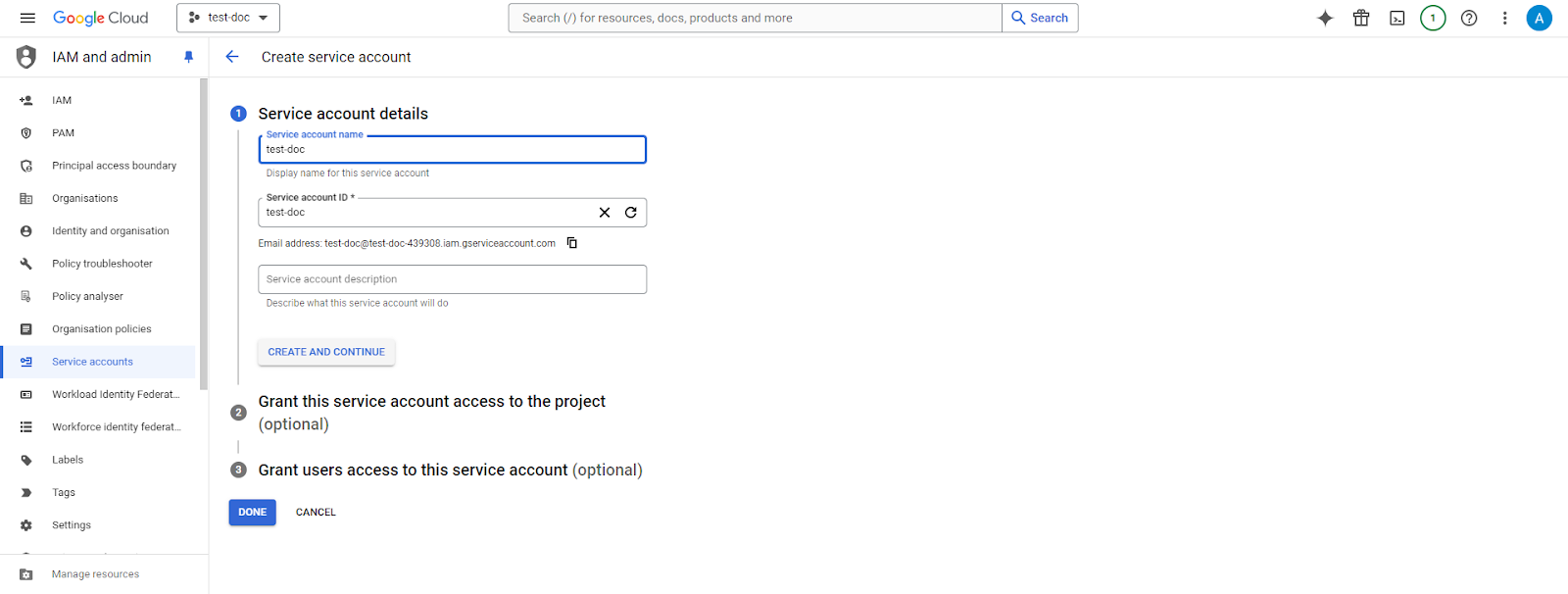
- Assign the role Project > Editor.
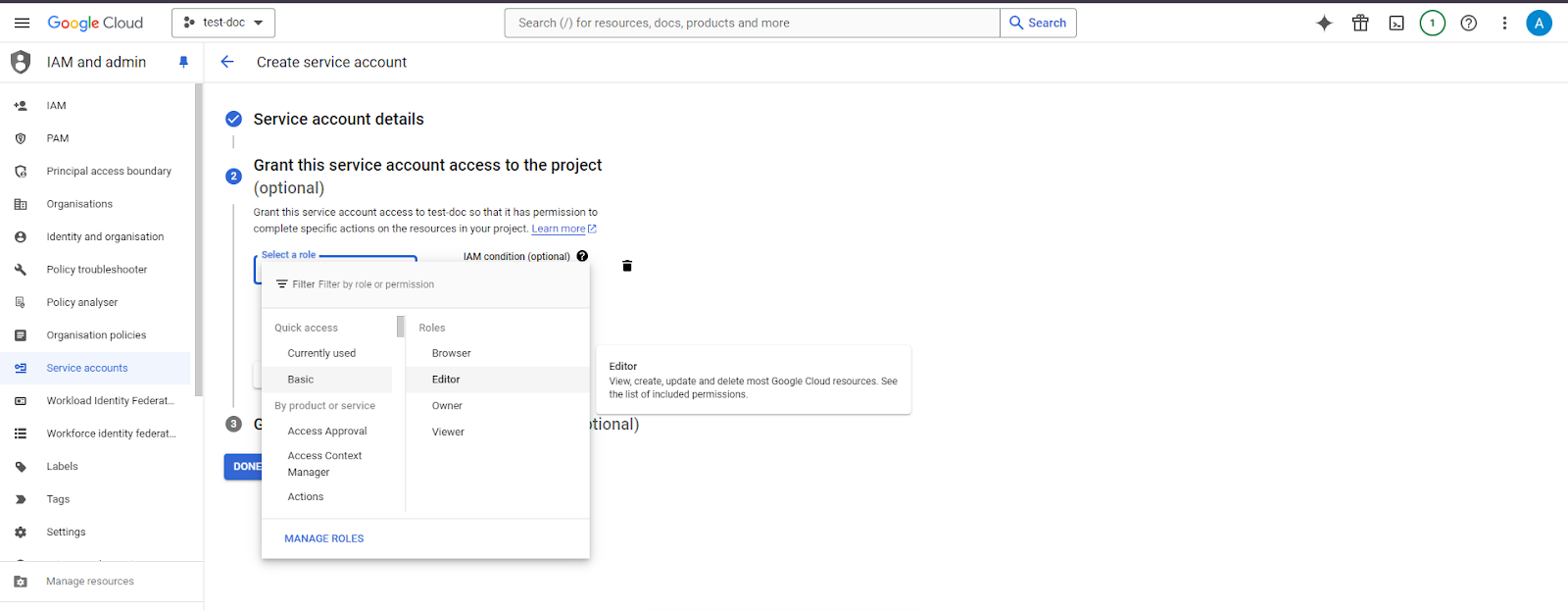
- Click Done to create the service account.
- Download the Service Account KeyClick on the newly created service account.
- Go to Keys > Add Key > Create New Key.

- Choose JSON format and download the key file.
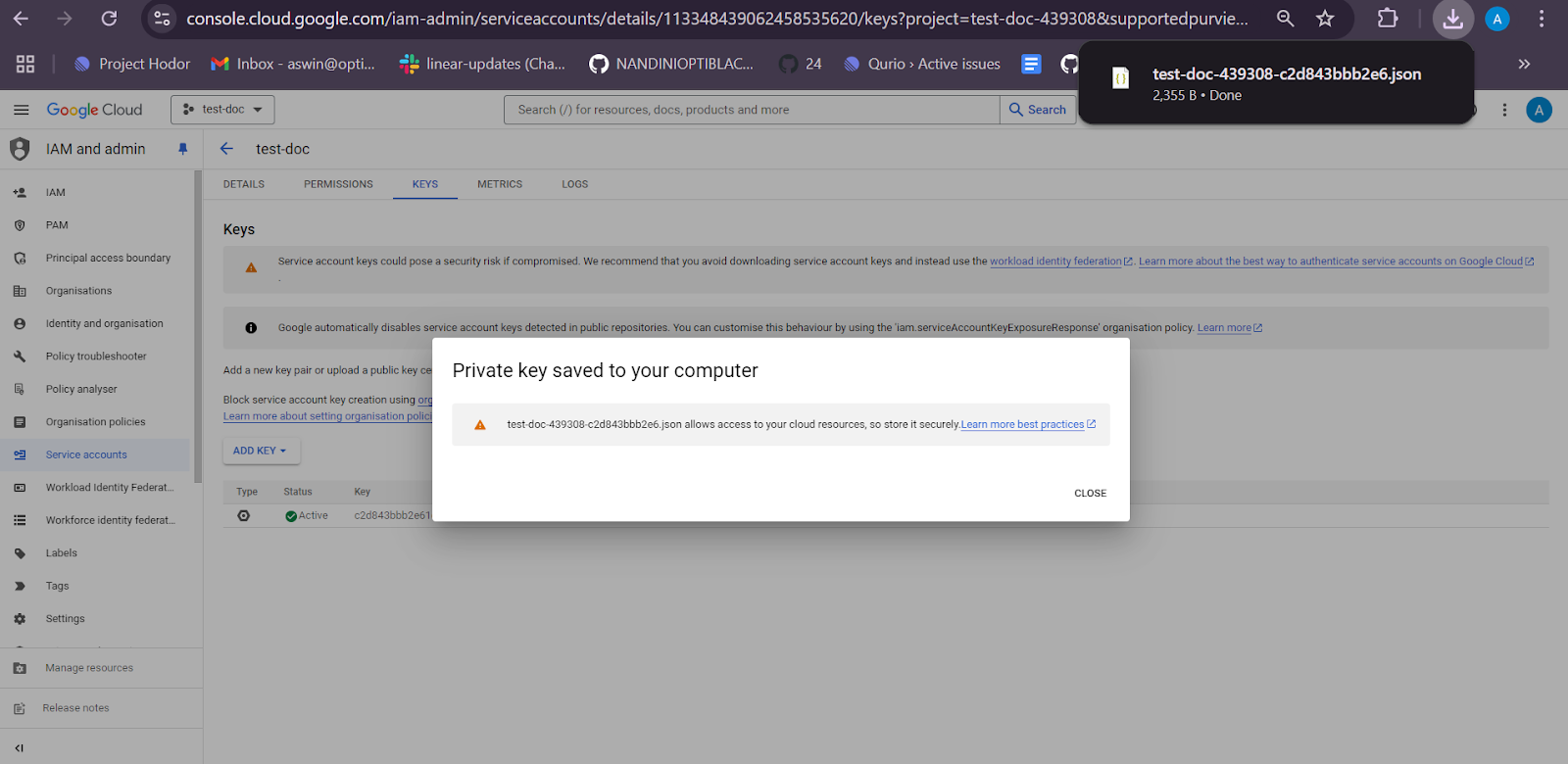
Step 3: Invite Service Account to Google Play Console
To enable the service account to access the Google Play Console, follow these steps:
- Open the Google Play Console (https://play.google.com/console).
- Navigate to Users and Permissions in the left navigation menu.
- Click Invite Users.
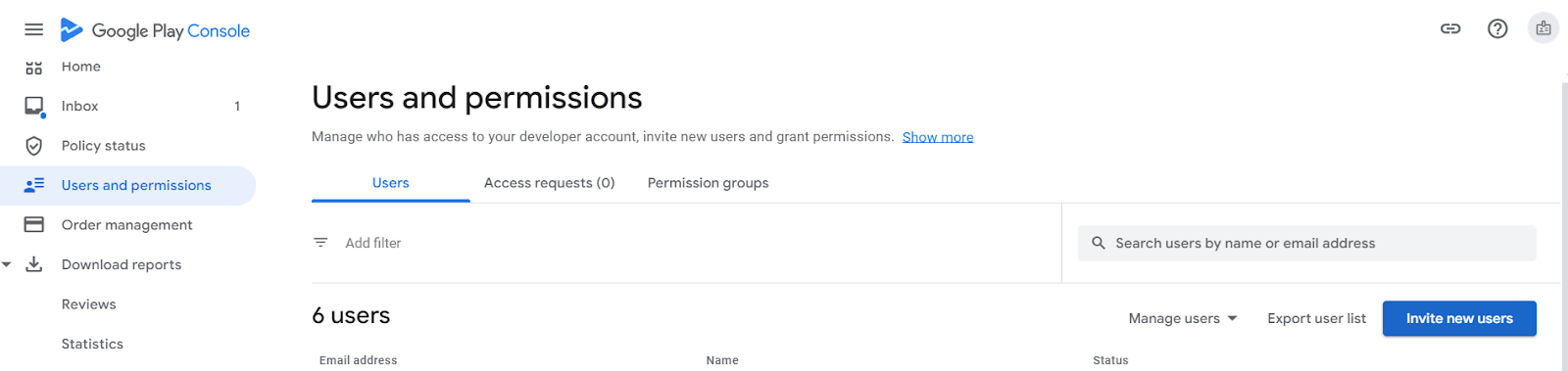
- Enter the service account email created earlier.
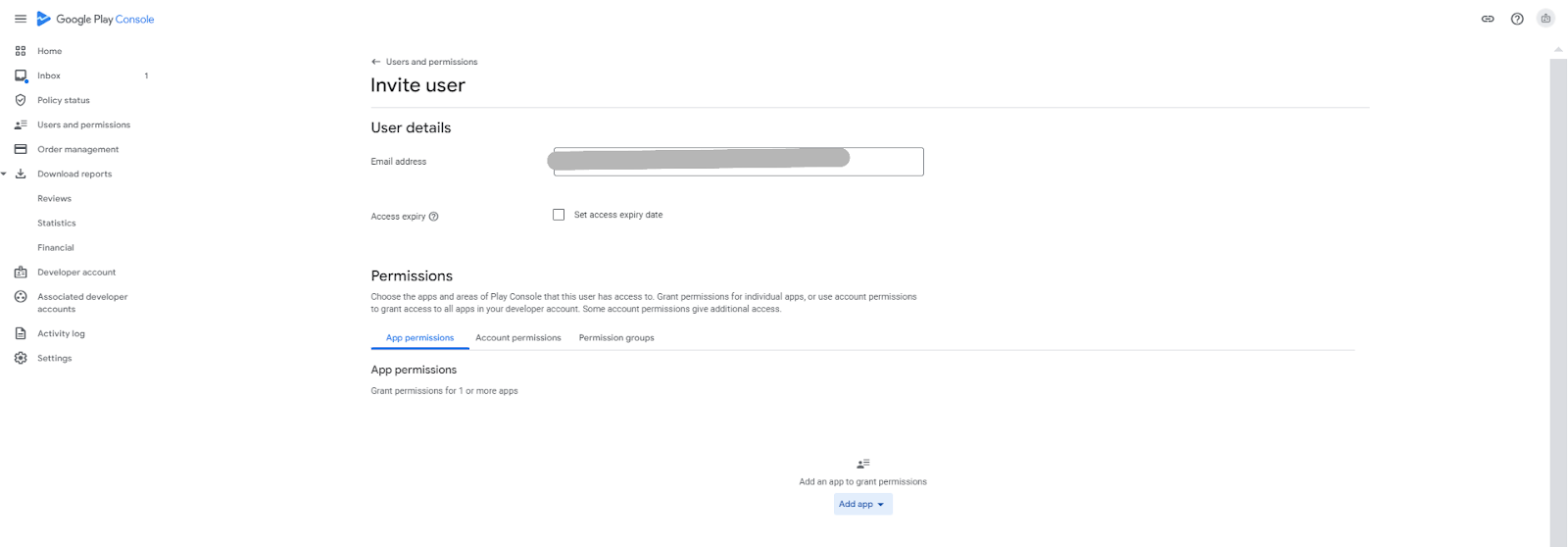
- Select the app you are working on.
- Assign the necessary permissions (e.g., View Financial Data and Manage Download Reports).
- Click on "Invite Users" and allow the necessary permissions
Note: The sync process can take up to 24 to 48 hours until the service account is fully integrated.
Step 4: Verify Files in Google Cloud Storage
Check the Files in the Storage Bucket:
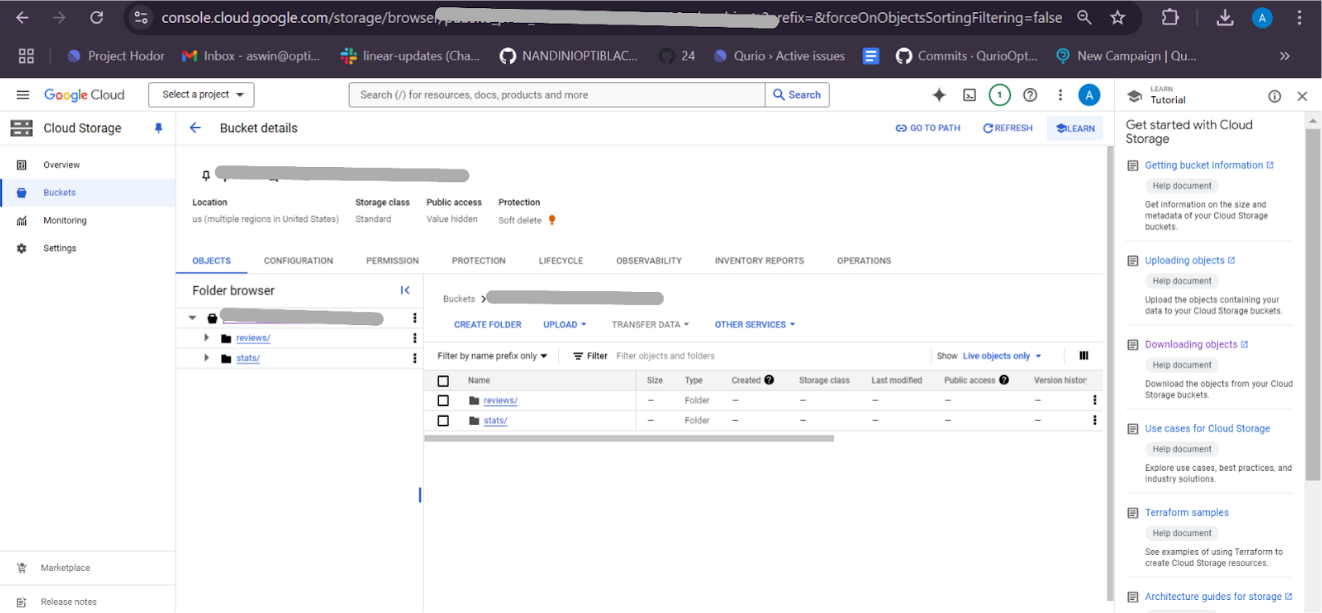
![]()
![]()
![]()
![]()
- Replace pubsite_prod_xx123456789 with your actual bucket name found on the Google Play Console under Download Reports > Statistics.

Identify the Required File: Locate reports like installs_com.exampleapp_202410_overview.csv (example name).
Step 5: Fetch Data from Google CloudStorage
- Set Up Node.js Environment:
- Create a new project directory:
- mkdir google-play-mixpanel
- cd google-play-mixpanel
- npm init -y
- Install Required Packages:
- npm install @google-cloud/storage dotenv
- Store your Google Cloud credentials in a .env file:
- GOOGLE_CLOUD_KEYFILE=path/to/your/service_account_key.json
Create a script to download the CSV file:
require('dotenv').config();
const { Storage } = require('@google-cloud/storage');
const path = require('path');
const storage = new Storage({
keyFilename: process.env.GOOGLE_
CLOUD_
KEYFILE,
});
const getAppStatsFile = async () => {
try {
const date = new Date();
const year = date.getFullYear().toString();
const month = (date.getMonth() + 1).toString().padStart(2,
'0');
const bucketName = 'pubsite
_prod
_
xx123456789'; // Replace with
your actual bucket name
const fileName=
`installs
_
com.exampleapp_${year}${month}_
overview.csv
`
;
const filePath =
`
stats/installs/${fileName}`
;
const destination = path.join(__
dirname,
`/reports/${fileName}`);
await storage.bucket(bucketName).file(filePath).download({
destination});
console.log('File downloaded successfully to:'
, destination);
return destination;}
catch (error) {
console.error('Error downloading file:'
, error.message);
}
};
getAppStatsFile();
Step 6: Parse the CSV Data
- Install the CSV Parser
- npm install csv-parser
Add Parsing Logic:
const fs = require('fs');
const csv = require('csv-parser');
const readCsvFile = async (filePath) => {
const results = [];
fs.createReadStream(filePath, { encoding: 'utf16le' })
.pipe(csv({ separator: '
'
,
, headers: true }))
.on('data'
, (row) => {
results.push(row);
})
.on('error'
, (error) => {
console.error('Error reading CSV file:'
, error.message);
})
.on('end'
, () => {
console.log('CSV Data Parsed:'
, results);
uploadToMixpanel(results); // Proceed with the Mixpanel
integration.
});
};
getAppStatsFile().then(readCsvFile);
Step 7: Set Up Mixpanel and Send Data
- Create a Mixpanel Account: Visit Mixpanel (https://mixpanel.com).
- Click Sign Up if you are new or Log In if you already have an account.
- Set Up a Project
- Click Create New Project from the Projects tab on your dashboard.
- Name your project, such as "Google Play Store Data Integration" and click
- Create Project.
- Retrieve Project Token and API Secret:
- Go to Settings > Project Settings under your project.
- Copy the Project Token.
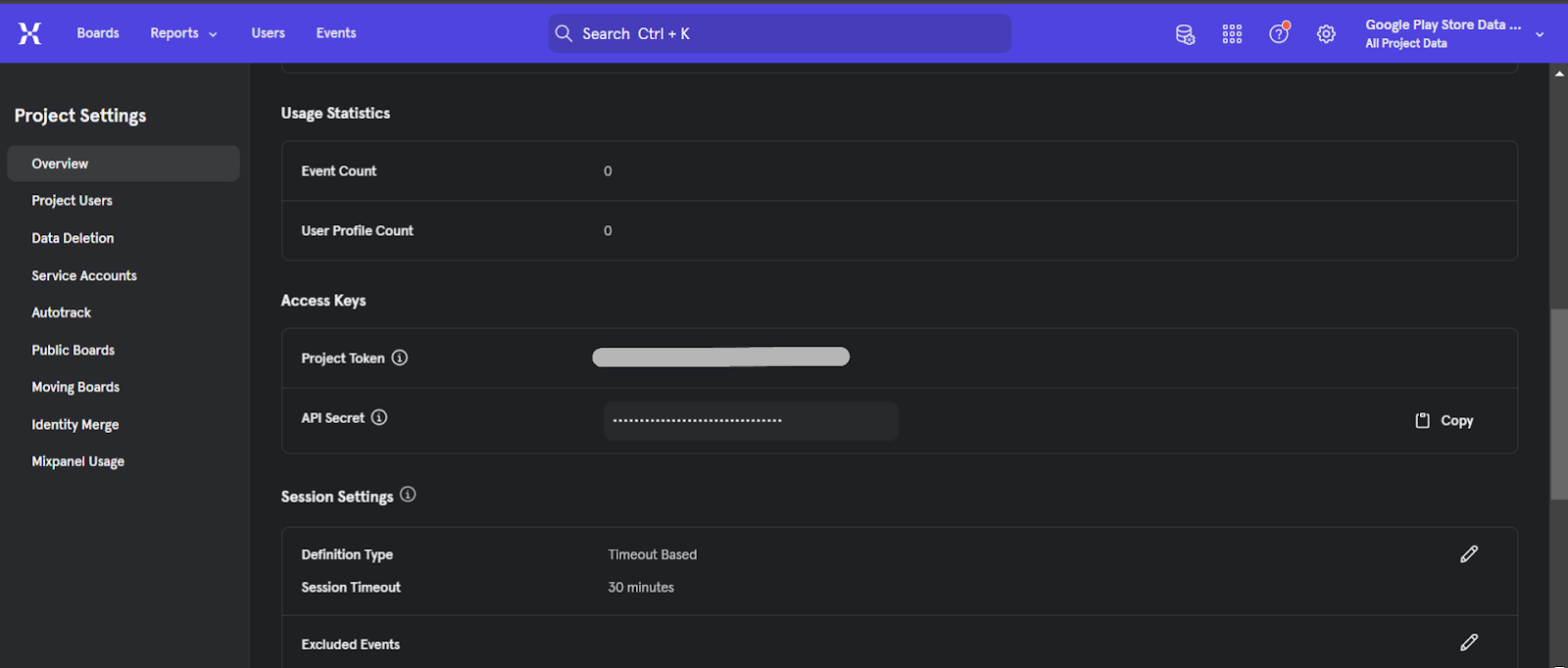
- Access the API Secret under Service Accounts if not already created.
- Store Mixpanel Credentials
- Add the following to your .env file:
MIXPANEL_
PROJECT
_
TOKEN=your
_project_
token
_
here
MIXPANEL
_
SECRET=your_
secret_
key_
here
- Install Mixpanel Library:
npm install mixpanel
- Write the Mixpanel Integration Script:
const mixpanel = require('mixpanel');
const mixpanelImporter =mixpanel.init(process.env.MIXPANEL PROJECT__TOKEN, {secret: process.env.MIXPANEL_SECRET, });
const uploadToMixpanel = (data) => { data.forEach(item => { const eventName = 'Install Stats'; const dateStr = item['_0']; // Adjust according to your CSV structure.
const time = new Date(dateStr).getTime();
const properties = {time: time, platform: 'android', activeDeviceInstalls: item['_8'], };
mixpanelImporter.import(eventName, time, properties, (err) =>
{ if (err) {
console.error(`Error sending event to Mixpanel: ${err}`);}
else { console.log(`Event tracked successfully: ${eventName}`, properties);}
}); }); };
getAppStatsFile().then(readCsvFile);
Additional Notes
- Modify Report Type: Change the fileName in the script to download different types of reports, such as reviews or statistics.
- Monitor Data in Mixpanel: Visit the Mixpanel dashboard (https://mixpanel.com) to visualize and analyze the tracked events.
- Google Cloud Documentation: Refer to Google Cloud Storage Documentation (https://cloud.google.com/storage/docs) for managing buckets and files.
- Mixpanel API Documentation: Check out the Mixpanel Developer Documentation (https://developer.mixpanel.com) for details on how to use the API.
Conclusion
By following this guide, you can automate the process of extracting Google Play Store data, parsing it into a usable format, and integrating it with Mixpanel for deeper insights. This setup allows you to gain valuable analytics, enabling data-driven decisions for your app's success.
Here is the output
how it looks
.png?width=2856&height=1636&name=image%20(11).png)